Sending HTTP headers with cURL
When working with web APIs or making complex web requests, including additional HTTP headers in your cURL commands is essential. These headers give the server critical context about the request, such as specifying content type, providing authentication credentials, or including custom data. For developers, understanding how to manipulate these headers is crucial to ensure that the server processes their requests correctly and efficiently. This guide will explore how to effectively use and customize HTTP headers in cURL requests to optimize your interactions with web servers.
How to send HTTP headers with cURL?
To send HTTP headers with cURL, you can use the -H or --header option followed by the header you want to include. A typical cURL request with headers might look like this:
curl -X GET "https://api.example.com/resource" \-H "Authorization: Bearer YOUR_ACCESS_TOKEN" \-H "Content-Type: application/json"
These headers provide the server with essential context, such as what kind of content to expect (Content-Type) and how to authenticate the request (Authorization).
What are default cURL headers?
By default, cURL does not include any special headers in its HTTP requests besides those automatically set by the HTTP protocol. The most common default headers that cURL might send are:
- User-Agent. cURL sends a User-Agent header that identifies the client software, usually in the form of "curl/" followed by the version number (e.g., "curl/7.69.0");
- Host. This header specifies the domain name of the server you're trying to reach. It's automatically set based on the URL provided in the request;
- Accept. By default, cURL sends "Accept: */*", indicating that the client is willing to accept any response.
These default headers provide basic information that helps the server understand and process the request. They can be overridden or supplemented with additional headers using various header options with cURL.
How to send custom headers with cURL?
To add a custom header to a cURL request, use the -H or --header flag followed by the header name and value:
curl -H "Content-Type: application/json" -H "X-Custom-Header: Value" http://example.com/api/resource
- Content-Type: application/json tells the server that the body of the request contains JSON data;
- X-Custom-Header: Value is an example of a custom header that could be used for application-specific purposes.
How to pass multiple headers with cURL?
To pass multiple headers with a cURL request, you can simply use the -H or --header flag multiple times, each followed by a different header name and value. This allows you to include various types of information in your request. Here’s an example:
curl -H "Content-Type: application/json" \-H "Authorization: Bearer YOUR_ACCESS_TOKEN" \-H "X-Custom-Header: Value" \http://example.com/api/resource
- Content-Type: application/json specifies that the request body is in JSON format;
- Authorization: Bearer YOUR_ACCESS_TOKEN adds an authorization token for secure API access;
- X-Custom-Header: Value demonstrates including an additional custom header for specific application needs.
How to show HTTP response headers with cURL?
To display the HTTP response headers when making a cURL request, you can use the -i or --include flag. This flag tells cURL to include the response headers in the output, allowing you to see important information such as status codes, server details, and cookies:
curl -i http://example.com/api/resource
Here’s an example of how the response would look like:
HTTP/1.1 200 OKDate: Tue, 27 Aug 2024 12:34:56 GMTContent-Type: application/jsonContent-Length: 123Connection: keep-aliveServer: Apache/2.4.41 (Ubuntu)X-Powered-By: PHP/7.4.3Set-Cookie: sessionid=abc123; Path=/; HttpOnly{"id": 1,"name": "Example Resource","status": "active"}
The response body follows these headers containing details about the requested resource.
How to print both request and response headers?
For debugging, seeing what's happening when sending a request with cURL might be helpful.
To have curl display the HTTP headers for both the request and the response, along with the response body and additional information provided by curl, use the -v or --verbose flag. Here's a basic example:
curl -v http://example.com/api/resource
The -v flag instructs cURL to print detailed information about the request and response, including the full set of HTTP headers.
Common use cases for cURL headers
Even though there are many possible use cases for cURL headers, here are a few of the most popular examples of HTTP headers in cURL requests.
Sending authenticated requests
When interacting with secured resources, you might need to include an authorization header. This header often carries tokens or credentials needed to authenticate the request:
curl -H "Authorization: Bearer YOUR_ACCESS_TOKEN" http://example.com/api/protected/resource
In this example, the Authorization header carries a bearer token, a common method used in OAuth2 authentication.
Changing the response format
The Accept header tells the server the type of content you’re willing to receive in response. This is crucial for APIs that can return data in multiple formats:
curl -H "Accept: application/xml" http://example.com/api/resource
Here, the request specifies that it prefers to receive XML data in response.
Specifying the User-Agent
Some servers return different data based on the client's User-Agent string. You can customize this header to mimic different devices or browsers:
curl -H "User-Agent: Mozilla/5.0 (iPhone; CPU iPhone OS 10_3 like Mac OS X) AppleWebKit/603.1.30 (KHTML, like Gecko) Version/10.0 Mobile/14E5239e Safari/602.1" http://example.com
This can be particularly useful for testing how your API responds to requests from different browsers or devices.
Sending the Content-Length header
While cURL automatically calculates and adds the Content-Length header, there might be cases where you need to override this behavior, especially when dealing with servers that have specific requirements:
curl -H "Content-Length: 0" http://example.com/api/empty-post
Customizing the Connection header
You can control aspects of the HTTP connection itself using headers like Connection: keep-alive or Connection: close, although such cases are rare since cURL and the server usually manage connection behavior efficiently:
curl -H "Connection: close" http://example.com/api/resource
Final notes
Effectively using cURL to send and manage HTTP headers is essential for interacting with web APIs and making complex web requests. By understanding various ways how to use headers, developers can ensure their requests are correctly formatted and fully understood by the server. Mastering these techniques allows for more precise control over the interaction between the client and the server, ultimately leading to more efficient and secure API communication.
About the author
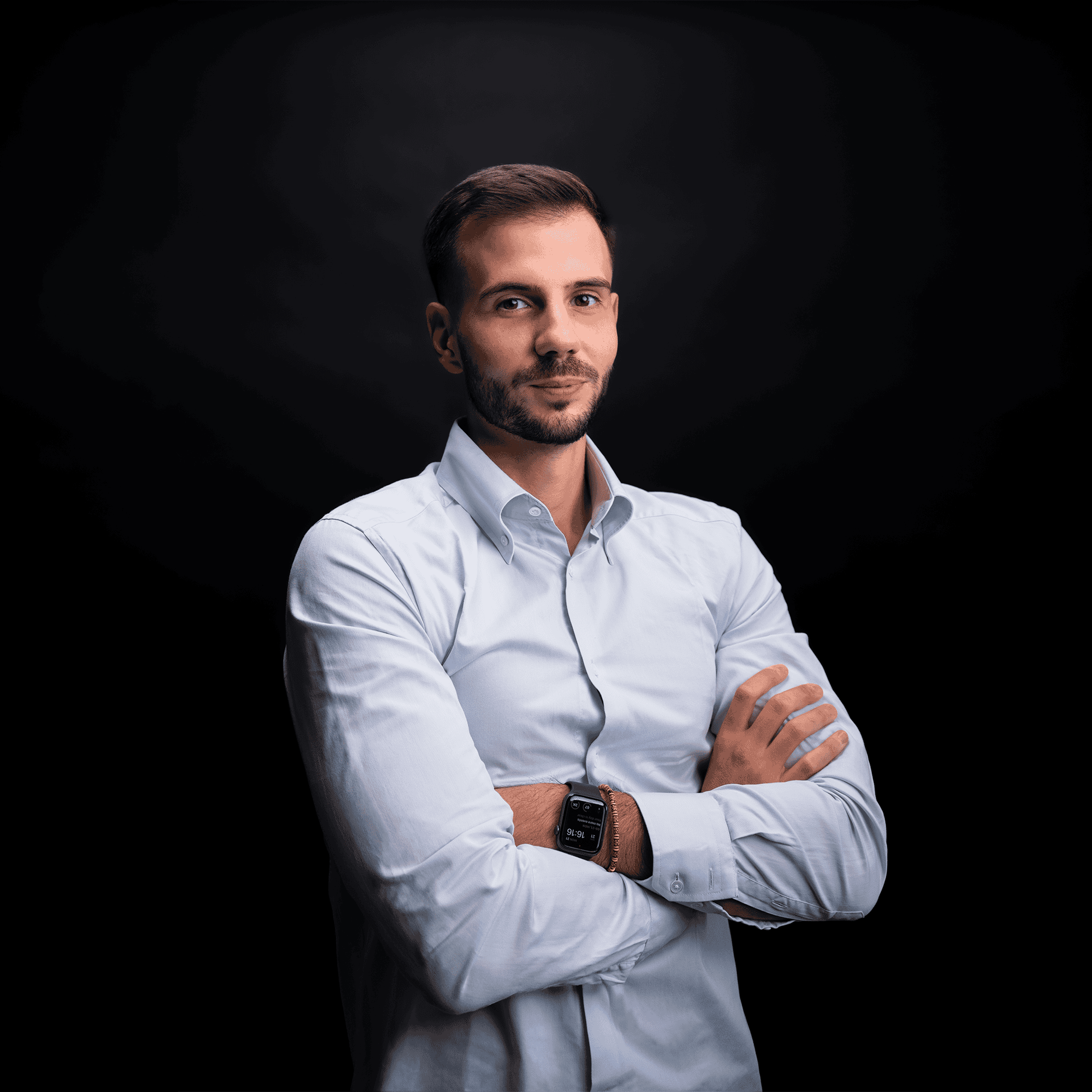
Martin Ganchev
VP Enterprise Partnerships
Martin, aka the driving force behind our business expansion, is extremely passionate about exploring fresh opportunities, fostering lasting relationships in the proxy market, and, of course, sharing his insights with you.
All information on Smartproxy Blog is provided on an "as is" basis and for informational purposes only. We make no representation and disclaim all liability with respect to your use of any information contained on Smartproxy Blog or any third-party websites that may be linked therein.